Tech
How to code a program that detects AI?
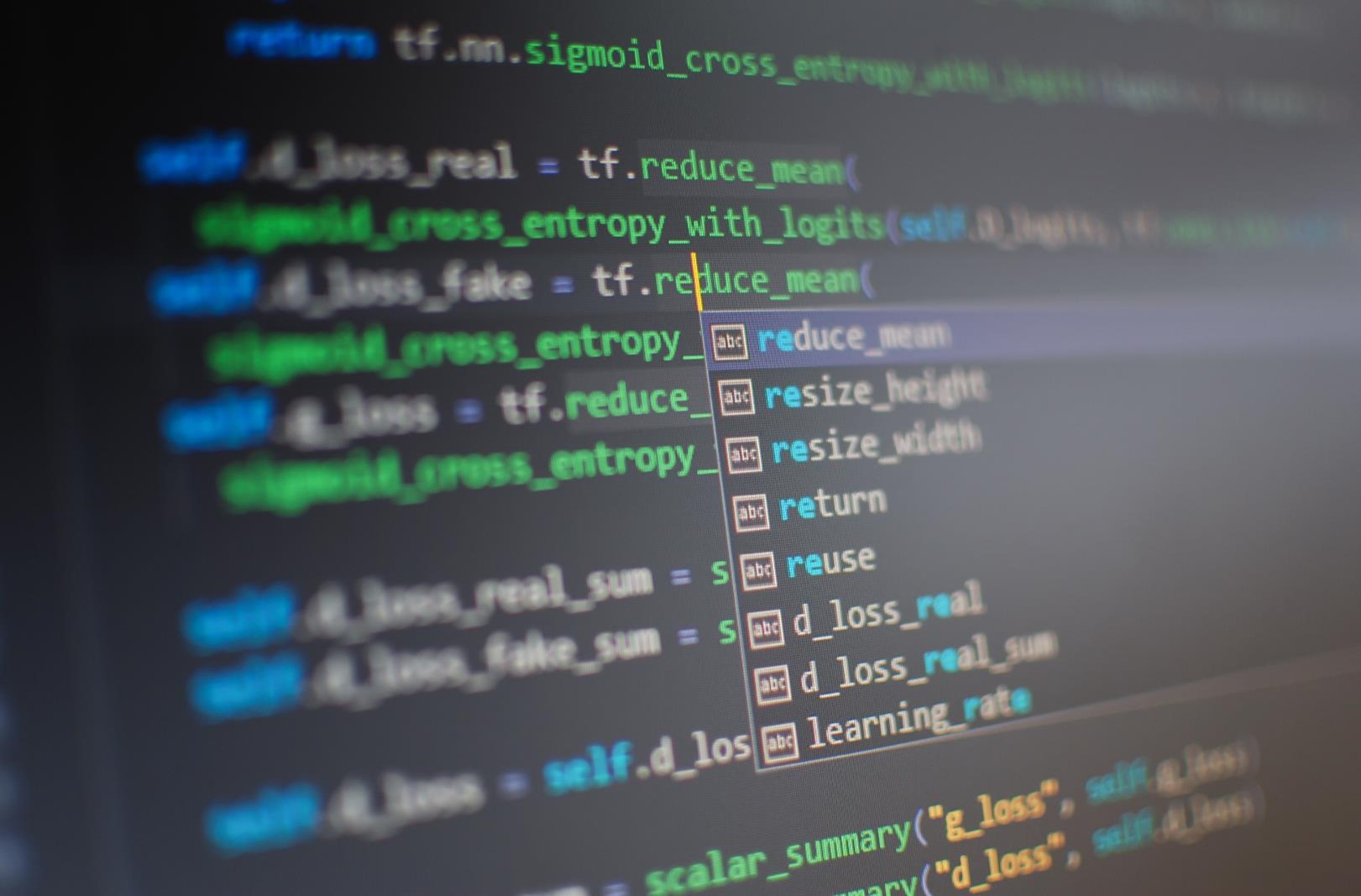
Detecting AI-generated content presents unique challenges due to the rapid advancement of technology and AI models, which make distinguishing outputs increasingly complex. Balancing false positives and negatives is critical to ensure accuracy without overcompensating.
Ethical considerations must be prioritized to respect privacy and ensure the responsible use of detection tools. Continuous updates and retraining are essential to keep up with evolving AI technologies.
Why detecting AI is important
The rapid evolution of AI has introduced challenges such as misinformation, fake media, and automated content that can be difficult to distinguish from human-generated work.
Developing a detection program helps address these challenges by providing tools for verification and accountability.
Applications of AI detection
- Plagiarism detection: Identifying AI-generated text in academic or creative work.
- Cybersecurity: Detecting automated bots in systems or online platforms.
- Media integrity: Verifying the authenticity of images or videos.
- Gaming: Ensuring fair play by detecting AI-assisted cheating.
Key concepts for coding an AI detection program
Before diving into coding, it’s essential to understand the foundational concepts.
Machine learning models
Most AI-generated content is created using machine learning models like GPT or GANs. Detecting such content involves training another machine learning model to recognize patterns associated with AI-generated outputs.
Features of AI-generated content
- Text: Repetitive phrases, overuse of certain structures, or a lack of genuine randomness.
- Images: Artifacts, inconsistencies in textures, or unrealistic details.
- Behavior: Predictable actions or responses that differ from human variability.
Steps to code a program that detects AI
This section outlines the step-by-step process of coding a program to detect AI, from understanding datasets to deploying machine learning models.
1. Define the scope of detection
Identify the type of AI-generated content you aim to detect, such as text, images, or behavior. This decision shapes the tools and techniques you will use.
2. Collect and label data
Gather datasets containing examples of both human-generated and AI-generated content. Label the data accurately for training and testing purposes.
- Text detection: Use datasets like OpenAI’s GPT outputs or human-written articles.
- Image detection: Collect images and authentic photos from GAN-generated libraries.
- Behavior analysis: Analyze logs or activity patterns of bots versus humans.
3. Choose a programming language and framework
Select a language and framework suitable for machine learning tasks. Python is a popular choice due to its extensive libraries and community support.
Recommended libraries:
- TensorFlow or PyTorch: For building and training machine learning models.
- Scikit-learn: For preprocessing data and implementing simpler models.
- NLTK or spaCy: For text analysis.
- OpenCV: For image processing.
4. Preprocess the data
Prepare the data for analysis by cleaning, normalizing, and transforming it into a suitable format.
Text preprocessing:
- Tokenize sentences and words.
- Remove stop words and special characters.
- Convert text to numerical representations using embeddings like Word2Vec or BERT.
Image preprocessing:
- Resize images to a standard dimension.
- Normalize pixel values.
- Augment data to improve model robustness.
5. Build and train the detection model
Develop a machine learning model tailored to the type of detection required.
Example for text detection:
pythonCopy codefrom sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
# Load and split data
X_train, X_test, y_train, y_test = train_test_split(data['text'], data['label'], test_size=0.2)
# Convert text to numerical data
vectorizer = TfidfVectorizer(max_features=5000)
X_train_tfidf = vectorizer.fit_transform(X_train)
X_test_tfidf = vectorizer.transform(X_test)
# Train a classifier
model = RandomForestClassifier()
model.fit(X_train_tfidf, y_train)
# Evaluate the model
accuracy = model.score(X_test_tfidf, y_test)
print(f"Model Accuracy: {accuracy}")
Example for image detection:
pythonCopy codeimport tensorflow as tf
from tensorflow.keras import layers, models
# Build a convolutional neural network
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(128, 128, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(1, activation='sigmoid')
])
# Compile the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=10, validation_data=(test_images, test_labels))
6. Test the model
Evaluate the model’s performance using the test dataset. Analyze metrics such as accuracy, precision, recall, and F1 score to assess its effectiveness.
7. Deploy the program
Once the model performs well, integrate it into an application or platform.
- Web application: Use Flask or Django to create an interface for uploading and analyzing content.
- Standalone tool: Build a desktop application using Python or Java.
8. Regularly update and improve
As AI evolves, so should your detection program. Update the model with new data and retrain it to handle emerging patterns in AI-generated content.
Challenges in Detecting AI
Explore the hurdles in identifying AI-generated content, including rapid AI evolution, achieving detection accuracy, and addressing ethical considerations effectively.
The rapid evolution of AI
AI models are continuously improving, making it harder to distinguish their outputs from human-generated content.
Balancing false positives and negatives
Achieving a balance between detecting AI and avoiding false positives is challenging but critical for reliable results.
Ethical considerations
Ensure the detection program is used ethically and does not infringe on privacy or rights.
Final thoughts
Learning how to code a program that detects AI empowers developers to address challenges posed by AI-generated content. By leveraging machine learning models, preprocessing data, and regularly updating the detection program, it’s possible to create robust tools for identifying AI-generated text, images, and behavior.
This capability is increasingly valuable across industries, ensuring transparency and accountability in an AI-driven world.
-
News1 week ago
Every Wednesday, Journal News Deliveries Were Made
-
News1 week ago
Young People Disapproving of Trump’s Job Performance After Six Months — CBS News Analysis
-
News1 week ago
Trump Update: US Envoy to Evaluate Food Distribution Centers in Gaza, According to White House | US News
-
News1 week ago
MLB Trade Deadline: Live Updates, News, Transactions, and Rumors – Reds Acquire Ke’Bryan Hayes, Both Rogers Twins on the Move
-
Business1 week ago
Top Investment Opportunities to Watch in 2025
-
News1 week ago
EPA to Revoke Landmark “Endangerment Finding” Key to Regulating Greenhouse Gases
-
News7 days ago
Markets Decline Following Trump Tariffs Announcement and Jobs Report
-
Business1 week ago
Unlocking Crowdfunding: A Startup’s Guide to Success