Tech
How to fix rounding errors in Java: A comprehensive guide
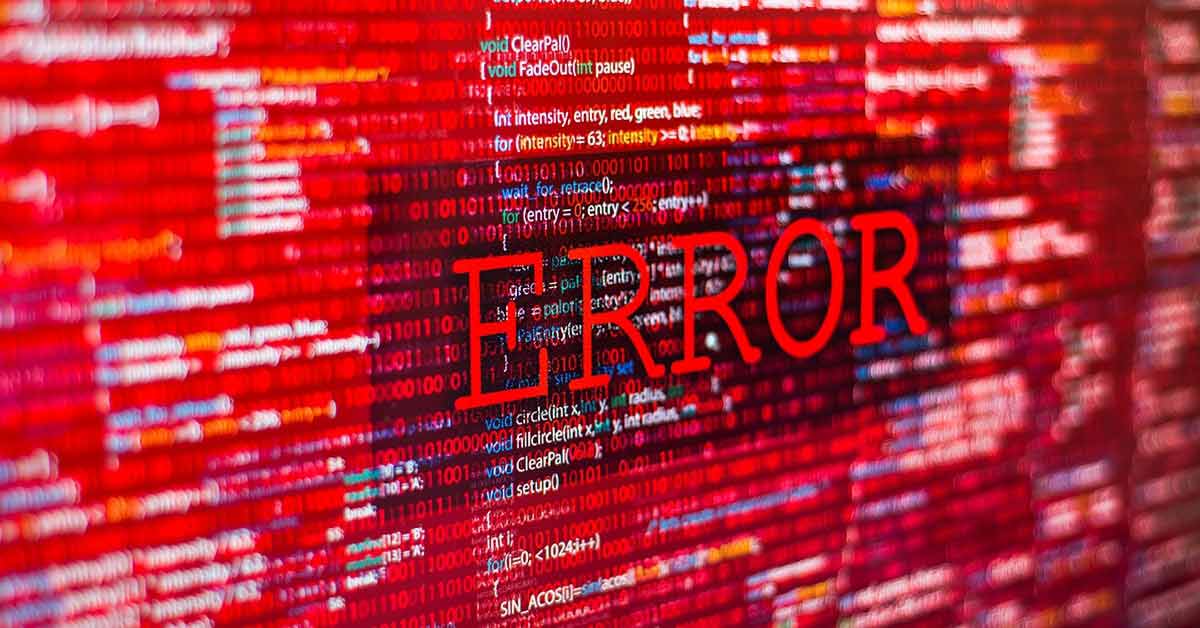
Rounding errors are a common issue in programming, particularly when working with floating-point arithmetic. In Java, these errors occur due to the way floating-point numbers are represented in memory.
Understanding how to fix rounding errors in Java is essential for ensuring accurate calculations in your applications, especially in fields like technology and finance.
This guide explores the causes of rounding errors and provides practical solutions to address them.
What are rounding errors?
Rounding errors in Java arise when the computer cannot precisely represent certain decimal numbers using binary floating-point format. This limitation leads to small inaccuracies in calculations, which can accumulate and impact the results.
Common causes of rounding errors
- Floating-point representation: Java uses the IEEE 754 standard for floating-point numbers, which can introduce small inaccuracies.
- Arithmetic operations: Repeated operations on floating-point numbers can amplify rounding errors.
- Precision loss: Converting between data types, such as from float to double, can result in precision loss.
Examples of rounding errors in Java
javaCopy codepublic class RoundingErrorExample {
public static void main(String[] args) {
double result = 0.1 + 0.2;
System.out.println(result); // Output: 0.30000000000000004
}
}
How to fix rounding errors in Java
Tips for fixing rounding errors in Java:
1. Use BigDecimal for precise calculations
The BigDecimal
class in Java provides precise control over decimal arithmetic and avoids rounding errors.
Example:
javaCopy codeimport java.math.BigDecimal;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal num1 = new BigDecimal("0.1");
BigDecimal num2 = new BigDecimal("0.2");
BigDecimal result = num1.add(num2);
System.out.println(result); // Output: 0.3
}
}
Tips for using BigDecimal:
- Always use
String
or BigDecimal.valueOf to avoid floating-point precision issues during initialization. - Use appropriate rounding modes when performing division with BigDecimal.
2. Use Math.round for simple rounding
For simple rounding tasks, the Math.round method can provide adequate results.
Example:
javaCopy codepublic class MathRoundExample {
public static void main(String[] args) {
double value = 0.34567;
double roundedValue = Math.round(value * 100.0) / 100.0;
System.out.println(roundedValue); // Output: 0.35
}
}
3. Format output using DecimalFormat
The DecimalFormat class allows you to format numbers to a specified decimal precision.
Example:
javaCopy codeimport java.text.DecimalFormat;
public class DecimalFormatExample {
public static void main(String[] args) {
double value = 0.34567;
DecimalFormat df = new DecimalFormat("#.##");
System.out.println(df.format(value)); // Output: 0.35
}
}
4. Use Integer Arithmetic for fixed-point calculations
When working with monetary values or scenarios requiring fixed precision, consider using integer arithmetic.
Example:
javaCopy codepublic class FixedPointExample {
public static void main(String[] args) {
int value1 = 10; // Representing $0.10
int value2 = 20; // Representing $0.20
int result = value1 + value2; // Result: 30 (Representing $0.30)
System.out.println("Result: $" + (result / 100.0)); // Output: $0.3
}
}
5. Avoid floating-point comparisons
Direct comparisons of floating-point numbers can lead to unexpected results due to precision issues. Instead, use a tolerance value.
Example:
javaCopy codepublic class FloatingPointComparison {
public static void main(String[] args) {
double num1 = 0.1 + 0.2;
double num2 = 0.3;
double epsilon = 0.00001;
if (Math.abs(num1 - num2) < epsilon) {
System.out.println("Numbers are equal");
} else {
System.out.println("Numbers are not equal");
}
}
}
6. Use proper rounding modes
When rounding is necessary, use proper rounding modes provided by BigDecimal.
Example:
javaCopy codeimport java.math.BigDecimal;
import java.math.RoundingMode;
public class RoundingModeExample {
public static void main(String[] args) {
BigDecimal value = new BigDecimal("2.345");
BigDecimal roundedValue = value.setScale(2, RoundingMode.HALF_UP);
System.out.println(roundedValue); // Output: 2.35
}
}
Best practices to minimize rounding errors
Minimizing rounding errors involves using precise tools like BigDecimal, avoiding mixed data types, and testing calculations with edge cases.
Use BigDecimal for high-precision needs
Always prefer BigDecimal for financial and high-precision applications to avoid floating-point errors.
Avoid mixing data types
Consistently use one data type for calculations to prevent precision loss during conversions.
Test with edge cases
Validate your program with edge cases that may trigger rounding errors.
Educate on floating-point limitations
Understanding the limitations of floating-point arithmetic can help write more robust code.
When to use floating-point numbers
Floating-point numbers are suitable for scenarios where minor precision errors are acceptable, such as scientific calculations or graphical simulations.
Final thoughts
Rounding errors in Java can significantly impact the accuracy of calculations, especially in critical applications. By leveraging tools like BigDecimal, Math.round, and DecimalFormat, and adhering to best practices, developers can minimize these errors effectively.
Understanding how to fix rounding errors in Java ensures precise and reliable results in your applications.